.:: Search-n-run ::.
Looks for new files and opens them
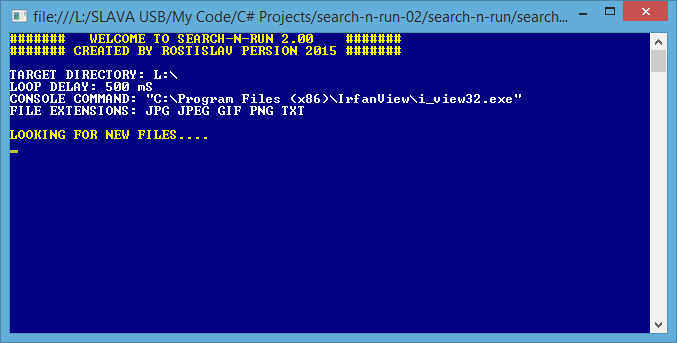
This is a program written in C# that monitors a folder for specified file extensions, and opens them when they are created.
SOFTWARE WRITTEN IN C#
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 | using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.IO; using System.Collections; using System.Threading; namespace search_n_run_02 { class Program { static void Main(string[] args) { // SET BACKGROUND COLOR Console.BackgroundColor = ConsoleColor.DarkBlue; Console.Clear(); // WELCOME MESSAGE Console.ForegroundColor = ConsoleColor.Yellow; Console.WriteLine("####### WELCOME TO SEARCH-N-RUN 2.00 #######"); Console.WriteLine("####### CREATED BY ROSTISLAV PERSION 2015 #######"); Console.WriteLine(""); // LOAD INI SETTINGS string targetDirectory = ""; int sleepTime = 0; string runCommand = ""; string[] fileExt = {}; try { // read from file System.IO.StreamReader file = new System.IO.StreamReader("args.ini"); string line1 = file.ReadLine(); string line2 = file.ReadLine(); string line3 = file.ReadLine(); string line4 = file.ReadLine(); file.Close(); // init vars targetDirectory = line1.Trim(); Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("TARGET DIRECTORY: " + targetDirectory); sleepTime = Convert.ToInt32(line2.Trim()); Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("LOOP DELAY: " + sleepTime.ToString().Trim() + " mS"); runCommand = line3.Trim(); Console.ForegroundColor = ConsoleColor.White; Console.WriteLine("CONSOLE COMMAND: " + runCommand); fileExt = line4.Split (','); Console.ForegroundColor = ConsoleColor.White; Console.Write("FILE EXTENSIONS: "); for (int i = 0; i < fileExt.Length; i++) { Console.Write(fileExt[i].ToUpper().Trim() + " "); } Console.WriteLine(); } catch (Exception e) { Console.ForegroundColor = ConsoleColor.Red; Console.WriteLine("PROBLEM LOADING SETTINGS!"); Console.ReadLine(); Environment.Exit(0); } // LOOK FOR NEW FILES Console.ForegroundColor = ConsoleColor.Yellow; Console.WriteLine(""); Console.WriteLine("LOOKING FOR NEW FILES...."); Console.WriteLine(""); Console.ForegroundColor = ConsoleColor.White; DateTime startTime = DateTime.Now; while (true) { string[] fileEntries = { }; try { fileEntries = Directory.GetFiles(targetDirectory); } catch(Exception e) { Console.ForegroundColor = ConsoleColor.Red; Console.WriteLine("PROBLEM READING FOLDER!"); Console.ReadLine(); Environment.Exit(0); } foreach (string fileName in fileEntries) { //IF FILE PATTERN THEN CONTINUE string[] fileParts = fileName.Split('.'); string ext = fileParts[fileParts.Length - 1].ToUpper().Trim(); bool isExt = false; for (int i = 0; i < fileExt.Length; i++) { if (ext.Trim().ToUpper() == fileExt[i].Trim().ToUpper()) { isExt = true; break; } } if(isExt == true) { DateTime creationTime = File.GetCreationTime(fileName); DateTime writeTime = File.GetLastWriteTime(fileName); if (creationTime < writeTime) { creationTime = writeTime; } if (startTime < creationTime) { Console.WriteLine("NEW FILE: " + fileName); System.Diagnostics.Process.Start(runCommand, fileName); } } } startTime = DateTime.Now; Thread.Sleep(sleepTime); } } } } |