.:: Distributed MD5 Cracker ::.
Distributed MD5 Cracker (PHP and now PYTHON)

This program is a brute force MD5 hash cracker. It generates strings and encodes them. Then it compares the encoding to the MD5 hash. If the encoding is the same as the hash, the program displays the result.
SOFTWARE WRITTEN IN PYTHON (USES POTENTIAL KEYWORDS)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 | import hashlib import math from datetime import datetime from posixpath import split #INIT INPUT mdhash = "46b8eb4802e02efeeee4770d73c37bfd" numstart = 0 numend = 88000000 #SETTINGS use_num = True use_alpha_lower = False use_alpha_upper = False potential_keywords = "Password,Love,Box,#" #PRINT HEADER print("########## INPUT ###########") print("MD5 HASH: " + str(mdhash)) print("START INDEX: " + str(numstart)) print("END INDEX: " + str(numend)) print("") #BUILD LEX lex = [" "] + potential_keywords.split(',') nums_list = ["0", "1", "2", "3", "4", "5", "6", "7", "8", "9"] alpha_low_list = ["a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z"] alpha_high_list = ["A","B","C","D","E","F","G","H","I","J","K","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z"] if use_num == True: lex += nums_list if use_alpha_lower == True: lex += alpha_low_list if use_alpha_upper == True: lex += alpha_high_list #PRINT DATE-TIME dateTimeObj = datetime.now() timestampStr = dateTimeObj.strftime("%m/%d/%Y, %H:%M:%S") print("########## SYSTEM ##########") print("STATUS: CRACKING MD5 HASH") #SHOW LEXICON LIST print("LEXICON: " + str(lex)) crack_method = "brute_force" if len(potential_keywords) > 0: crack_method += "_keywords" if use_num == True: crack_method += "_numbers" if use_alpha_lower == True: crack_method += "_lower_alpha" if use_alpha_upper == True: crack_method += "_upper_alpha" print("METHOD: " + crack_method) print("START TIMESTAMP: " + timestampStr) print("") numbase = len(lex) res = 0 powres = 0 mant = 0 num = 0 num2 = 0 found = False num2 = numstart while num2 < numend: num2 = num2 + 1 num = num2 mant = math.floor(math.log(num,numbase)) strres = "" while mant >= 0: powres = pow(numbase, mant) res = math.floor(num / powres) strres = strres + lex[res] num = num - math.floor(res * powres) mant = mant - 1 #GENERATE MD5 FROM TRY md1 = hashlib.md5(strres.encode()) md2 = md1.hexdigest() #CHECK TO SEE IF TRY IS SAME AS INPUT if md2 == mdhash: #PRINT FOOTER, FOUND dateTimeObj = datetime.now() timestampStr = dateTimeObj.strftime("%m/%d/%Y, %H:%M:%S") print("########## RESULT ##########") print("STATUS: FOUND") print("RESULT: " + strres) print("END TIMESTAMP: " + timestampStr) found = True if found == True: break #PRINT FOOTER, NOT FOUND if found == False: dateTimeObj = datetime.now() timestampStr = dateTimeObj.strftime("%m/%d/%Y, %H:%M:%S") print("########## RESULT ##########") print("STATUS: NOT FOUND") print("END TIMESTAMP: " + timestampStr) print() input("Press ENTER to exit...") |
SOFTWARE WRITTEN IN PHP
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 | <?php //get user input $mdhash = "76175b4492147d59fa0376a44bf9ead7"; //$_GET['mdhash']; $numstart = 0; //$_GET['start']; $numend = 8800000; //$_GET['end']; $iffoundemail = "seeplusplus@gmail.com"; //$_GET['email']; //show user input print("\n<input>\n"); print(" <md5hash>" . $mdhash . "</md5hash>\n"); print(" <startvalue>" . $numstart . "</startvalue>\n"); print(" <endvalue>" . $numend . "</endvalue>\n"); print("</input>\n"); //show system settings print("\n<system>\n"); print(" <date>" . date("d/m/y", time()) . "</date>\n"); print(" <time>" . date("H:i:s", time()) . "</time>\n"); print(" <method>brute_force_ABC_abc_123</method>\n"); print("</system>\n\n"); //define character set $lex = Array( " ","a","b","c","d","e","f","g","h","i","j","k","l", "m","n","o","p","q","r","s","t","u","v","w","x","y", "z","A","B","C","D","E","F","G","H","I","J","K","L", "M","N","O","P","Q","R","S","T","U","V","W","X","Y", "Z","0","1","2","3","4","5","6","7","8","9"); //count character set $numbase = count($lex); //declare working variables $res=0; $powres=0; $mant=0; $num=0; $num2=0;$found=false; //main loop $t1 = time(); for ($num2=$numstart;$num2<$numend;$num2++) { $num = $num2; $mant = floor(log($num,$numbase)); $strres = ""; while ($mant >= 0) { $powres = pow($numbase, $mant); $res = floor($num / $powres); $strres .= $lex[$res]; $num -= floor($res * $powres); $mant--; } if (md5($strres) == $mdhash) { $found = true; break; } } $t2 = time(); //show result if ($found) { print("<output>\n"); print(" <cputime>" . ($t2 - $t1) . "</cputime>\n"); print(" <ascii>" . $strres . "</ascii>\n"); print("</output>\n"); } else { print("<output>\n"); print(" <cputime>" . ($t2 - $t1) . "</cputime>\n"); print("</output>\n"); } ?> |
03/15/2020 - PORTED TO PYTHON
MD5 CRACKER PYTHON CODE
CLICK HERE TO DOWNLOAD
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 | import hashlib import math from datetime import datetime #INIT INPUT mdhash = "9491876179d7a80bb5c86f15dbe31422" numstart = 0 numend = 88000000 #PRINT HEADER print("########## INPUT ###########") print("MD5 HASH: " + str(mdhash)) print("START INDEX: " + str(numstart)) print("END INDEX: " + str(numend)) print("") #PRINT DATE-TIME dateTimeObj = datetime.now() timestampStr = dateTimeObj.strftime("%m/%d/%Y, %H:%M:%S") print("########## SYSTEM ##########") print("STATUS: CRACKING MD5 HASH") print("METHOD: brute_force_ABC_abc_123") print("START TIMESTAMP: " + timestampStr) print("") #DEFINE LEX lex = [" ","a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z","A","B","C","D","E","F","G","H","I","J","K","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z","0","1","2","3","4","5","6","7","8","9"] numbase = len(lex) res = 0 powres = 0 mant = 0 num = 0 num2 = 0 found = False num2 = numstart while num2 < numend: num2 = num2 + 1 num = num2 mant = math.floor(math.log(num,numbase)) strres = "" while mant >= 0: powres = pow(numbase, mant) res = math.floor(num / powres) strres = strres + lex[res] num = num - math.floor(res * powres) mant = mant - 1 #GENERATE MD5 FROM TRY md1 = hashlib.md5(strres.encode()) md2 = md1.hexdigest() #CHECK TO SEE IF TRY IS SAME AS INPUT if md2 == mdhash: #PRINT FOOTER, FOUND dateTimeObj = datetime.now() timestampStr = dateTimeObj.strftime("%m/%d/%Y, %H:%M:%S") print("########## RESULT ##########") print("STATUS: FOUND") print("RESULT: " + strres) print("END TIMESTAMP: " + timestampStr) found = True if found == True: break #PRINT FOOTER, NOT FOUND if found == False: dateTimeObj = datetime.now() timestampStr = dateTimeObj.strftime("%m/%d/%Y, %H:%M:%S") print("########## RESULT ##########") print("STATUS: NOT FOUND") print("END TIMESTAMP: " + timestampStr) print() input("Press ENTER to exit...") |
MD5 CRACKER PYTHON CODE (GUI)
CLICK HERE TO DOWNLOAD (GUI)
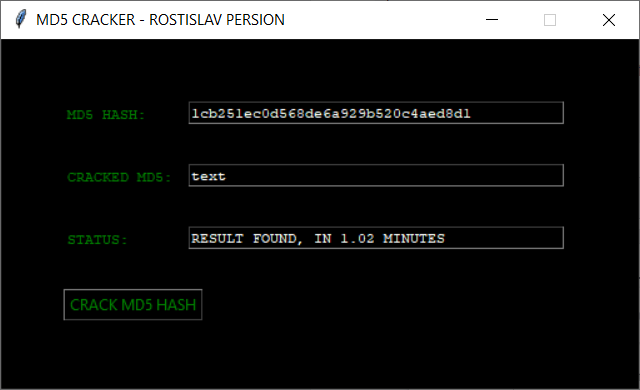
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 | from tkinter import * import hashlib import math from datetime import datetime import logging import threading import time from timeit import default_timer as timer def crack(): ######## DISABLE BUTTON b1.config(state="disabled") # SET CRACKING t3.delete(0, END) t3.insert(END, "CRACKING MD5...") # CLEAR OLD RESULT t2.delete(0, END) #GET MD5 VALUE mdhash = t1.get().strip() #START CODE numstart = 0 numend = 1000000000000 #2147483647 #DEFINE LEX lex = [" ","a","b","c","d","e","f","g","h","i","j","k","l","m","n","o","p","q","r","s","t","u","v","w","x","y","z","A","B","C","D","E","F","G","H","I","J","K","L","M","N","O","P","Q","R","S","T","U","V","W","X","Y","Z","0","1","2","3","4","5","6","7","8","9"] # GET LEX SIZE numbase = len(lex) # INIT VARS res = 0 powres = 0 mant = 0 num = 0 num2 = 0 found = False #TIME SPAN STUFF start = timer() # MAIN LOOP num2 = numstart while num2 < numend: num2 = num2 + 1 num = num2 mant = math.floor(math.log(num,numbase)) strres = "" while mant >= 0: powres = pow(numbase, mant) res = math.floor(num / powres) strres = strres + lex[res] num = num - math.floor(res * powres) mant = mant - 1 #GENERATE MD5 FROM TRY md1 = hashlib.md5(strres.encode()) md2 = md1.hexdigest() #CHECK TO SEE IF TRY IS SAME AS INPUT if md2 == mdhash: #PRINT FOOTER, FOUND #TIME SPAN STUFF end = timer() time_dif = round((end - start) / 60,2) t3.delete(0, END) t3.insert(END, "RESULT FOUND, IN " + str(time_dif) + " MINUTES") t2.delete(0, END) t2.insert(END, strres) found = True if found == True: break #PRINT FOOTER, NOT FOUND if found == False: t3.delete(0, END) t3.insert(END, "RESULT NOT FOUND!") t2.delete(0, END) t2.insert(END, strres) ######## ENABLE BUTTON b1.config(state="normal") def runCrack(): thread1 = threading.Thread(target=crack) thread1.start() win = Tk() win.title("MD5 CRACKER - ROSTISLAV PERSION") win.geometry("510x280") win.configure(background='black') win.resizable(0, 0) # CREATE LABELS lbl1=Label(win, text='MD5 HASH:',fg = "green",bg = "black",font=("Courier", 8)) lbl2=Label(win, text='CRACKED MD5:',fg = "green",bg = "black",font=("Courier", 8)) lbl3=Label(win, text='STATUS:',fg = "green",bg = "black",font=("Courier", 8)) # CREATE TEXT BOXES t1=Entry() t1.configure(background='black',foreground='white',font=("Courier", 8)) t1.insert(END,"033bd94b1168d7e4f0d644c3c95e35bf") t2=Entry() t2.configure(background='black',foreground='white',font=("Courier", 8)) t3=Entry() t3.configure(background='black',foreground='white',font=("Courier", 8)) # PLACE FIRST lbl1.place(x=50, y=50) t1.place(x=150, y=50, width=300) # PLACE SECOND lbl2.place(x=50, y=100) t2.place(x=150, y=100, width=300) # PLACE THIRD lbl3.place(x=50, y=150) t3.place(x=150, y=150, width=300) # BUTTON STUFF b1=Button(win, text='CRACK MD5 HASH', command=runCrack) b1.configure(background='black',foreground='green',font=("Courier", 8)) b1.place(x=50, y=200) # SET READY STATUS t3.delete(0, END) t3.insert(END, "READY...") win.mainloop() |