.:: Geolocation ::.
Finding city, state and country from IP
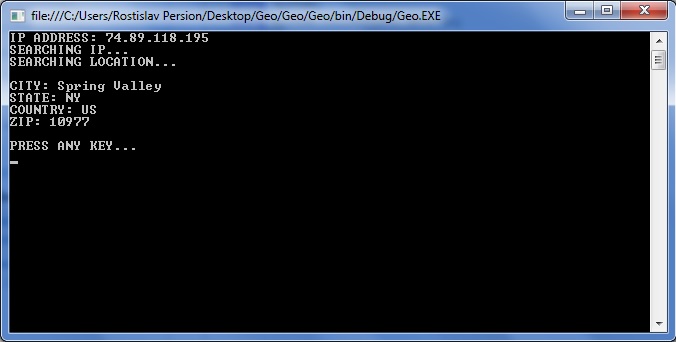
This is a program that outputs your location based on your IP address. The C# program uses two CSV files to achieve this. The first file contains a range of IP addresses with a location code on the same line. Once you find your IP range, you will find the location code (IP addresses are in long integer format). The second file contains location codes as the primary key, along with city, state, country, zip, metro code and area code.
EXECUTABLE
CSV DATABASE #1 (IP-START, IP-END, LOCATION-CODE)
CSV DATABASE #2 (LOCATION-CODE, COUNTRY, STATE, CITY, ZIP, LAT, LON, METRO-CODE, AREA-CODE)
SOFTWARE WRITTEN IN C#
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 | using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Net; using System.IO; namespace Geo { class Program { static long IPToInt(string addr) { return (long) (uint) IPAddress.NetworkToHostOrder((int) IPAddress.Parse(addr).Address); } static void Main(string[] args) { // get and convert input Console.Write("IP ADDRESS: "); string ip_string = Console.ReadLine(); long ip_long = IPToInt(ip_string); // Read the block file and display it line by line. Console.WriteLine("SEARCHING IP..."); long location_code = 0; string line = ""; StreamReader file = new StreamReader(@"GeoLiteCity-Blocks.csv"); while ((line = file.ReadLine()) != null) { string[] parts = line.Split(','); long ip1 = Convert.ToInt64(parts[0].Trim('"')); long ip2 = Convert.ToInt64(parts[1].Trim('"')); long location = Convert.ToInt64(parts[2].Trim('"')); if ((ip_long >= ip1) && (ip_long <= ip2)) { location_code = location; break; } } file.Close(); // Read the location file and display it line by line. Console.WriteLine("SEARCHING LOCATION..."); string line2 = ""; StreamReader file2 = new StreamReader(@"GeoLiteCity-Location.csv"); while ((line2 = file2.ReadLine()) != null) { string[] parts2 = line2.Split(','); long location2 = Convert.ToInt64(parts2[0].Trim('"')); string country = parts2[1].Trim('"'); string state = parts2[2].Trim('"'); string city = parts2[3].Trim('"'); string zip = parts2[4].Trim('"'); if (location2 == location_code) { Console.WriteLine(); Console.WriteLine("CITY: " + city); Console.WriteLine("STATE: " + state); Console.WriteLine("COUNTRY: " + country); Console.WriteLine("ZIP: " + zip); Console.WriteLine(); } } file.Close(); Console.WriteLine("PRESS ANY KEY..."); Console.ReadLine(); } } } |